Thursday, June 17, 2010
Weekly Report 9
This was the final week before our demo. We have tested the application over and over again and removed bugs. My duty was to test the Android part. We, Çiğdem and I, found some bugs in Gallery part and fixed them. We prepared some scenarios too for both image processing part and Google Maps part.
Weekly Report 9
This week, I obtained the relevant images from the database and passed to the image processing part by means of various mysql queries. After compeleting the project me and Guliz tested the product and cleaned the bugs. We prepared scenarios for the final demo and filled the database with images ,their locations and features of them. We are glad to say that we finalized our project, enjoyed a lot while developing it and learned many issues about groupwork, image processing and Android and those were invaluable experiences.
Weekly Report 8
This week I prepared the features of the image processing part so as to insert them to the database. In this way, we were not going to process each image in the database when we do the similarity check using the picture received from the server. That was a challenging process, because I was not able to find an example of saving opencv features into the database on internet, documentaations or manuals of openCV. There were two critical structures called cvSeq and cvMat and unlike cvSeq, the cvMat, which corresponds to a matrix, will easily be saved as an xml document. But cvSeq was collecting objects where cvMat was a union of float double and other basic types. Therefore, converting one into another was not easy to figure out. Finally I decided to divide cvSeq's properties into different cvMat objects and then saving those cvMat matrices into different xml files. Also recovered those xmls to cvSeq's by first converting them to different cvMat matrices and recovering old cvSeq objects by merging those various cvMat matrices. I prepared two different programs, one for preparing xml files and other for recovering cvSeq objects. And I integrated the xml to cvSeq part into the image processing module in order to speed up the application.
Weekly Report 7
This week I tried to integrate the image processing module to the project, after testing the surf method in-depth. Since image processing should be as fast as possible, I implemented it in c++ using opencv library. But I implemented various parts of the server side in java for the sake of simplicity. Therefore, I had two options during integration process:
- using java native api
- calling the c++ program as a process
Weekly Report 8
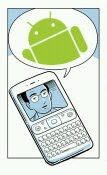
This week I worked on implementing the camera functionality of our product. Simulating the camera was a bit hard, but in the end I was able to do it in a proper way successfully.
First we have to make things clear. Android does not use the web cam to simulate the camera but when you correctly implemented it, you will get a default image from the platform and this will prove us that we are on the right way. First we have to give permission to the application to use camera functionality. We do it by adding “
Then we will get a camera from the system.
mCamera = Camera.open();
We will set the parameters of the camera:
Camera.Parameters params = mCamera.getParameters();
mSize = params.getPictureSize();
params.setPictureFormat(PixelFormat.JPEG);
mCamera.setParameters(params);
Then, we have to implement the callback function of the camera:
Camera.PictureCallback photoCallback = new Camera.PictureCallback() {
public void onPictureTaken(byte[] data, Camera camera) {
writeTextOnPicture(data);
}
};
With the above function we will get the default picture as a byte array. We will convert it to Bitmap object then will set the view to that image and we are done.
Weekly Report 7
This week I worked on sending MMS feature of our product. Actually, sending MMS is easy to implement.
Uri mmsUri = Uri.parse("file://"+"/sdcard" + IMAGE_FOLDER + this.pictureName + EXTENTION);
Intent intent = new Intent(Intent.ACTION_SEND);
intent.putExtra("sms_body", "Hi how are you");
intent.putExtra(Intent.EXTRA_STREAM, mmsUri);
intent.setType("image/png");
startActivity(intent);
We will add the picture we want to send as MMS and for that we need the content URI of the file. After that we will add a text to the MMS. However, Android emulator does not support sending MMS option. Normally, we were to type the port number of another emulator inside the “to” part of our emulator and the message would be sent immediately to the other emulator. I tested it and saw that it really doesn't work. However I was able to send SMS message to the other emulator when I deleted the image from the MMS message. This proved that the code I implemented is correct.
Uri mmsUri = Uri.parse("file://"+"/sdcard" + IMAGE_FOLDER + this.pictureName + EXTENTION);
Intent intent = new Intent(Intent.ACTION_SEND);
intent.putExtra("sms_body", "Hi how are you");
intent.putExtra(Intent.EXTRA_STREAM, mmsUri);
intent.setType("image/png");
startActivity(intent);
We will add the picture we want to send as MMS and for that we need the content URI of the file. After that we will add a text to the MMS. However, Android emulator does not support sending MMS option. Normally, we were to type the port number of another emulator inside the “to” part of our emulator and the message would be sent immediately to the other emulator. I tested it and saw that it really doesn't work. However I was able to send SMS message to the other emulator when I deleted the image from the MMS message. This proved that the code I implemented is correct.
Weekly Report 6
This week, I worked on extracting the name of a place from Google Maps by using the GPS information. For that we have to first extract the longitude and latitude of the location in a proper way.
public void extractGPS() {
LocationManager lm = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
Location location = lm
.getLastKnownLocation(LocationManager.GPS_PROVIDER);
if (location != null) {
latitude = location.getLatitude();
longitude = location.getLongitude();
}
lm.requestLocationUpdates(LocationManager.GPS_PROVIDER, 1000L, 500.0f,
this);
}
With that code, we will get the GPS information from the emulator. After that we can get the name of the landmark. We start with giving permission to our application to use the Google Maps API by adding those lines to the android manifest:
First we get a geocoder object.
Geocoder geocoder = new Geocoder(this, Locale.getDefault());
Then, we will extract the address for our current location by using the geocode object.
List addresses = geocoder.getFromLocation(latitude,longitude, 1);
We will get the first line of the address, the rest will be discarded.
Address address = addresses.get(0);
Finally, we will extract the most meaningful part of that adress.
address.getFeatureName();
That's it. That way, we will get the name of the landmark.
public void extractGPS() {
LocationManager lm = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
Location location = lm
.getLastKnownLocation(LocationManager.GPS_PROVIDER);
if (location != null) {
latitude = location.getLatitude();
longitude = location.getLongitude();
}
lm.requestLocationUpdates(LocationManager.GPS_PROVIDER, 1000L, 500.0f,
this);
}
With that code, we will get the GPS information from the emulator. After that we can get the name of the landmark. We start with giving permission to our application to use the Google Maps API by adding those lines to the android manifest:
First we get a geocoder object.
Geocoder geocoder = new Geocoder(this, Locale.getDefault());
Then, we will extract the address for our current location by using the geocode object.
List addresses = geocoder.getFromLocation(latitude,longitude, 1);
We will get the first line of the address, the rest will be discarded.
Address address = addresses.get(0);
Finally, we will extract the most meaningful part of that adress.
address.getFeatureName();
That's it. That way, we will get the name of the landmark.
Subscribe to:
Posts (Atom)